how to check if character is double quote in js
- Authors
- Name
- Geeks Kai
- @KaiGeeks
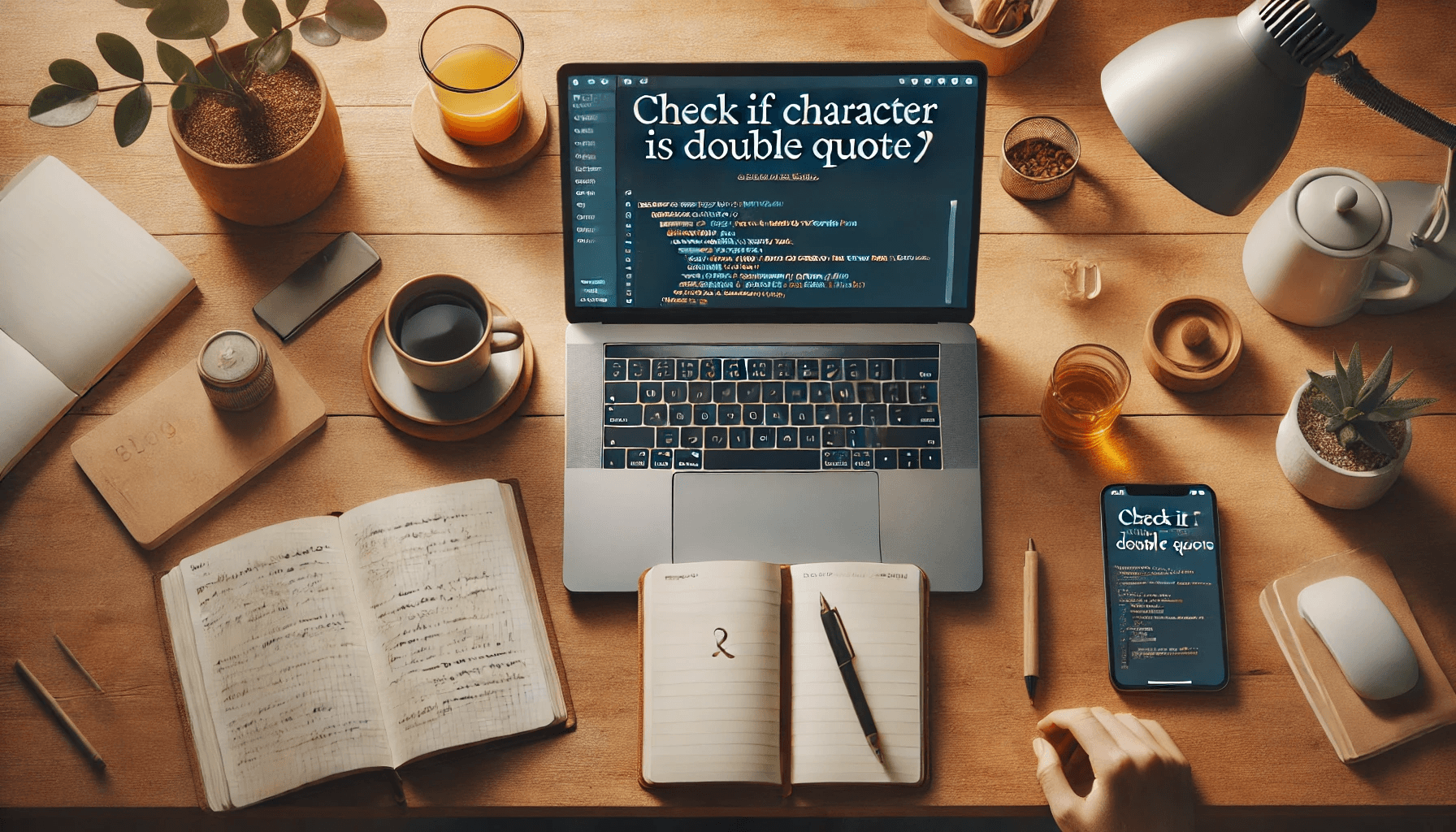
In JavaScript, a double quote ("
) is a common character used to denote strings. If you're working with strings or parsing text data, you may need to check if a character is a double quote. In this guide, we'll explore different methods to check if a character is a double quote in JavaScript.
1. Using Comparison Operators
One of the simplest ways to check if a character is a double quote is by using comparison operators. You can compare the character directly with the double quote character ("
).
const char = '"'
if (char === '"') {
console.log("The character is a double quote.")
} else {
console.log("The character is not a double quote.")
}
In this example, we compare the character char
with the double quote character ("
). If the character is a double quote, the message "The character is a double quote." is displayed; otherwise, the message "The character is not a double quote." is displayed.
charCodeAt()
Method
2. Using Another method to check if a character is a double quote is by using the charCodeAt()
method. This method returns the Unicode value of the character at a specified index in a string. The Unicode value of a double quote character ("
) is 34.
const char = '"'
if (char.charCodeAt(0) === 34) {
console.log("The character is a double quote.")
} else {
console.log("The character is not a double quote.")
}
In this example, we use the charCodeAt()
method to get the Unicode value of the character char
at index 0. We then compare this value with 34, which is the Unicode value of a double quote character. If the character is a double quote, the message "The character is a double quote." is displayed; otherwise, the message "The character is not a double quote." is displayed.
3. Using Regular Expressions
Regular expressions provide a powerful way to match patterns in strings, including specific characters like double quotes. You can use a regular expression to check if a character is a double quote.
const char = '"'
if (/"/.test(char)) {
console.log("The character is a double quote.")
} else {
console.log("The character is not a double quote.")
}
In this example, we use a regular expression /"/
to test if the character char
is a double quote. If the character is a double quote, the message "The character is a double quote." is displayed; otherwise, the message "The character is not a double quote." is displayed.
4. Using the match() method with a regular expression:
You can also use the match()
method with a regular expression to check if a character is a double quote.
const char = '"'
if (char.match(/"/)) {
console.log("The character is a double quote.")
} else {
console.log("The character is not a double quote.")
}
In this example, we use the match()
method with a regular expression /"/
to check if the character char
is a double quote. If the character is a double quote, the message "The character is a double quote." is displayed; otherwise, the message "The character is not a double quote." is displayed.
indexOf()
method
5. You can also use the indexOf()
method to check if a character is a double quote.
const char = '"'
if (char.indexOf('"') !== -1) {
console.log("The character is a double quote.")
} else {
console.log("The character is not a double quote.")
}
In this example, we use the indexOf()
method to check if the character char
contains a double quote. If the character is a double quote, the message "The character is a double quote." is displayed; otherwise, the message "The character is not a double quote." is displayed.
Summary
🔥 Using Comparison Operators:
- Directly compare the character with the double quote character (
"
).
- Directly compare the character with the double quote character (
🌟 Using
charCodeAt()
Method:- Get the Unicode value of the character and compare it with the Unicode value of a double quote.
🔄 Using Regular Expressions:
- Another approach suggested is using regex:
/['||"]/.test(str);
- 🔍 Using
indexOf
:
- A common method is to use
indexOf
to check for both quotes. - Example:
if (str.indexOf('\'') >= 0 && str.indexOf('"') >= 0) { //do something }
- 📜 Alternative Code Snippet:
- A similar solution is provided with a slight variation:
if((str.indexOf('\'') > -1) && (str.indexOf('"') > -1)) { //Code here }
Comparison
Method | Description |
---|---|
indexOf Method | Checks if both quotes exist using indexOf function. |
charCodeAt Method | Utilizes the charCodeAt method to check for the Unicode value of the character. |
Regular Expression | Utilizes regex to test for the presence of both quotes in the string. |
Alternative Snippet | Offers a slightly different implementation using indexOf . |