react dev only displays: Troubleshooting Rendering Issues
- Authors
- Name
- Geeks Kai
- @KaiGeeks
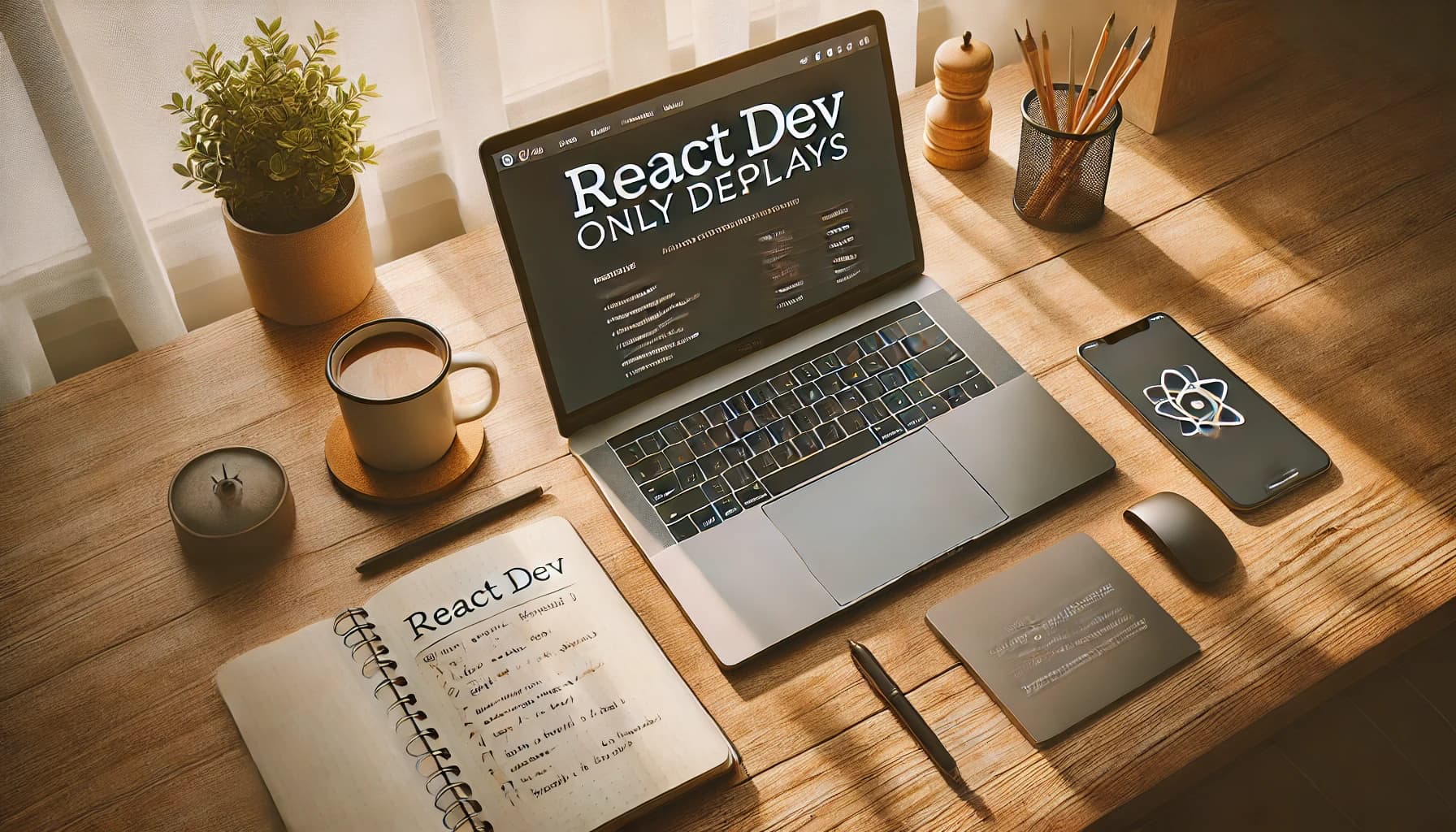
Understanding "react dev only displays" Challenges
When developers encounter the "React dev only displays" issue, it becomes a critical roadblock in the application development process. This complex problem manifests when React applications render only a fraction of the expected content, leaving developers frustrated and seeking comprehensive solutions.
Diagnostic Approach to Partial Rendering
1. Conditional Rendering Complexities
Conditional rendering is often the primary culprit behind incomplete displays. Consider this typical scenario:
function UserDashboard({ user }) {
// Potential rendering limitation
return user.isAdmin ? (
<div>
<AdminPanel />
<AdvancedReporting />
{user.hasSpecialAccess && <SuperAdminTools />}
</div>
) : null;
}
In this example, multiple nested conditions can lead to unexpected rendering behaviors. Each nested condition introduces a potential point of failure:
- If
user
is undefined - If
isAdmin
is false - If
hasSpecialAccess
is not explicitly handled
2. State Initialization and Management Challenges
Improper state management frequently causes partial rendering:
function DataVisualizationComponent() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
async function fetchData() {
try {
const result = await apiService.getData();
setData(result);
setLoading(false);
} catch (error) {
// Critical: Handle error state
setLoading(false);
setData([]);
}
}
fetchData();
}, []);
// Potential rendering issues
if (loading) return <Spinner />;
if (!data || data.length === 0) return <NoDataMessage />;
return <ComplexVisualization data={data} />;
}
Observations:
- Explicit error handling prevents "black hole" rendering
- Multiple state conditions control component visibility
- Proper loading and empty states are crucial
3. Asynchronous Data Fetching Pitfalls
Unresolved promises create significant rendering challenges:
function AsyncContentLoader() {
const [content, setContent] = useState({
articles: [],
user: null,
permissions: []
});
useEffect(() => {
Promise.all([
fetchArticles(),
fetchUserProfile(),
fetchPermissions()
]).then(([articles, user, permissions]) => {
setContent({ articles, user, permissions });
});
}, []);
// Complex conditional rendering
return (
<div>
{content.user && <UserHeader user={content.user} />}
{content.articles.length > 0 && <ArticleList articles={content.articles} />}
{content.permissions.includes('admin') && <AdminDashboard />}
</div>
);
}
Key Considerations:
- Parallel data fetching using
Promise.all()
- Granular rendering based on specific data availability
- Potential for partial or incomplete rendering
Advanced Debugging Strategies
React Developer Tools Techniques
Component Inspection
- Use React DevTools to examine component hierarchy
- Verify props and state at each rendering stage
- Identify components that aren't rendering as expected
Performance Profiling
- Record rendering performance
- Identify unnecessary re-renders
- Optimize component update mechanisms
Performance Optimization Approaches
Memoization Techniques
const MemoizedComponent = React.memo(ExpensiveComponent, (prevProps, nextProps) => { // Custom comparison logic return prevProps.criticalData === nextProps.criticalData; });
Code Splitting and Lazy Loading
const AdminPanel = React.lazy(() => import('./AdminPanel')); function App() { return ( <Suspense fallback={<LoadingIndicator />}> <AdminPanel /> </Suspense> ); }
Common Resolution Strategies
- Implement Comprehensive Error Boundaries
- Use Default Props and Values
- Leverage TypeScript for Stricter Type Checking
- Add Explicit Null and Undefined Checks
Conclusion
Resolving "React dev only displays" issues requires a multifaceted approach combining diagnostic skills, performance optimization, and robust error handling. By understanding the intricate rendering mechanisms and implementing strategic debugging techniques, developers can create more reliable and performant React applications.
Key Takeaways
- Conditional rendering requires careful, explicit logic
- State management is crucial for complete rendering
- Asynchronous operations demand comprehensive handling
- React DevTools are indispensable for thorough debugging