DeepSeek API Integration: Mastering the Process
- Authors
- Name
- Geeks Kai
- @KaiGeeks
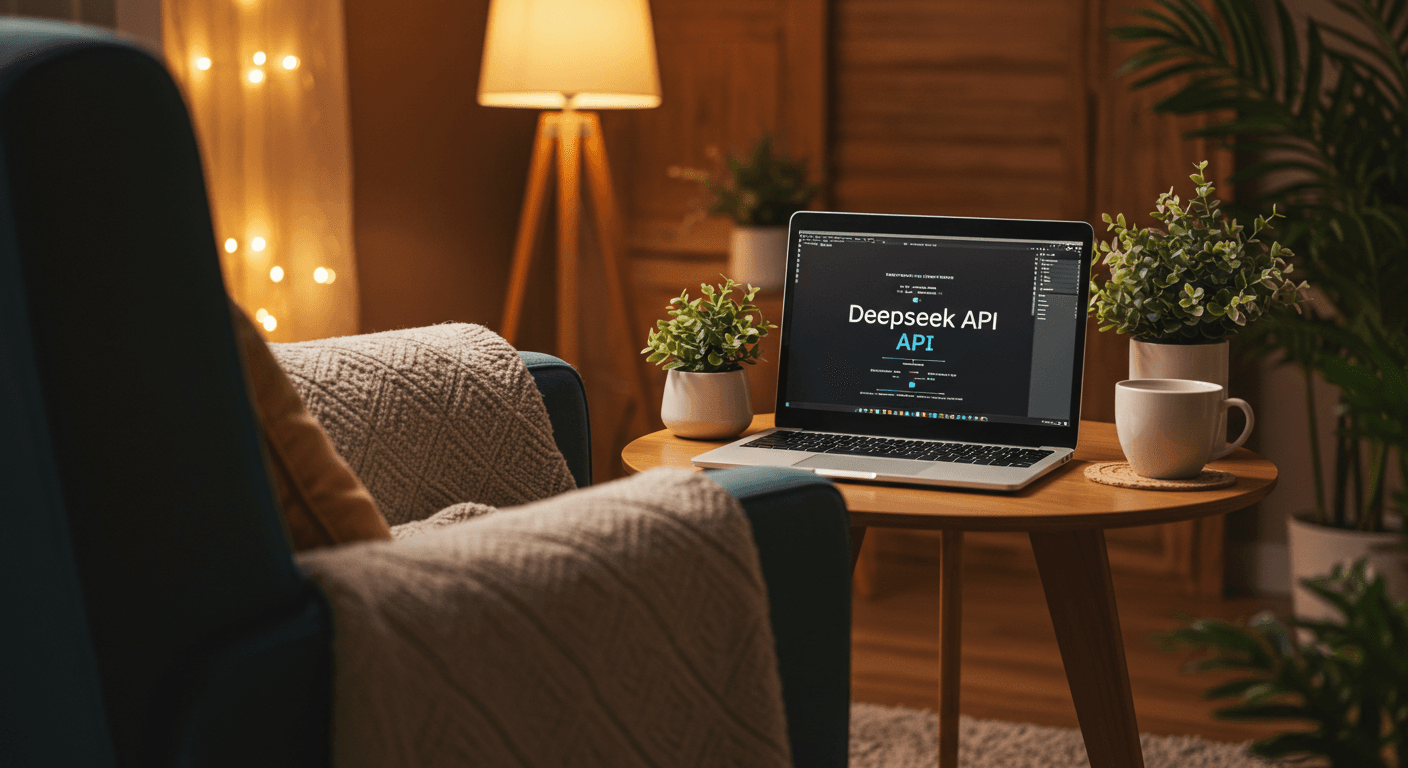
Key Highlights
- DeepSeek API makes it easy to use its advanced AI models in different applications.
- It has features that are similar to OpenAI's API, but it is much cheaper.
- DeepSeek R1, their top reasoning model, performs close to OpenAI's o1 on common tests.
- The platform includes a simple step-by-step guide for easy API integration.
- Developers can use DeepSeek API for many AI applications. This includes chatbots and complex reasoning systems.
- Even with its advantages, the DeepSeek API has been looked at closely for security issues and worries about censorship.
Introduction
The world of Artificial Intelligence is changing fast. DeepSeek API is a strong tool for developers who want to add smart AI features to their apps. With DeepSeek API, you can easily connect with DeepSeek's advanced AI models. This opens up many opportunities for creating smart AI applications.
Understanding the Basics of DeepSeek API
DeepSeek API makes it easy to use DeepSeek's strong AI models. These models are trained on a lot of data. They can understand and create text like a person, solve tough problems, and give insights from huge amounts of information.
The API works like a link between your app and the AI models from DeepSeek. By sending simple requests to the API, you can use the AI features you need and add them to your projects easily. This gives developers a lot of choices. They can build AI-powered functions without needing to start from the beginning.
Basic API Request Example
import requests
def make_deepseek_request(api_key, prompt):
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json'
}
data = {
'model': 'deepseek-r1',
'messages': [{'role': 'user', 'content': prompt}],
'stream': False
}
response = requests.post(
'https://api.deepseek.ai/v1/chat/completions',
headers=headers,
json=data
)
return response.json()
The Core Features of DeepSeek API
One great thing about the DeepSeek API is its wide range of features. It allows developers to have fine control over AI models with adjustable settings. This helps in fine-tuning responses and adapting behavior to fit specific needs of the application.
Configuration Parameters
Parameter | Description |
---|---|
base_url | DeepSeek API endpoint URL |
api_key | Your unique DeepSeek API key |
model | Specifies the DeepSeek model you want to use |
messages | An array of messages comprising the conversation history |
stream | Controls whether to receive a stream of responses(True/False) |
How DeepSeek API Enhances AI Applications
DeepSeek API can greatly change many AI applications. For example, it can run advanced AI chat models that can chat in a natural way and understand the context. This allows us to create new chatbots for customer support, virtual helpers, and more.
Streaming Response Example
import sseclient
def stream_deepseek_response(api_key, prompt):
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json',
'Accept': 'text/event-stream'
}
data = {
'model': 'deepseek-r1',
'messages': [{'role': 'user', 'content': prompt}],
'stream': True
}
response = requests.post(
'https://api.deepseek.ai/v1/chat/completions',
headers=headers,
json=data,
stream=True
)
client = sseclient.SSEClient(response)
for event in client.events():
yield event.data
Step-by-Step Guide to API Integration
Registering for a DeepSeek Account
- Go to the DeepSeek website
- Find the sign-up button
- Follow the instructions: Fill in your name, email address, and password
Generating and Managing Your API Keys
- Access the API Key Section: Log into your DeepSeek account and navigate to the API Key section
- Create a New API Key: Look for an option to "Create API Key"
- Store Your Key Securely: Copy and store the API Key safely
Error Handling Example
def handle_deepseek_request(api_key, prompt):
try:
response = make_deepseek_request(api_key, prompt)
if response.get('error'):
return {
'status': 'error',
'message': response['error']['message']
}
return {
'status': 'success',
'data': response['choices'][0]['message']['content']
}
except requests.exceptions.RequestException as e:
return {
'status': 'error',
'message': f'Network error: {str(e)}'
}
except Exception as e:
return {
'status': 'error',
'message': f'Unexpected error: {str(e)}'
}
Debugging Common Integration Issues
Common Issues and Solutions
Invalid API Key
- Check API key format
- Verify key hasn't expired
- Ensure proper key permissions
Rate Limiting
- Implement exponential backoff
- Monitor usage limits
- Consider upgrading plan if needed
Rate Limit Handler Example
import time
from functools import wraps
def rate_limit_handler(max_retries=3, delay=1):
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
retries = 0
while retries < max_retries:
try:
return func(*args, **kwargs)
except RateLimitException:
retries += 1
if retries == max_retries:
raise
time.sleep(delay * (2 ** retries))
return None
return wrapper
return decorator
Frequently Asked Questions
How Do I Reset My DeepSeek API Key?
You can reset your DeepSeek API Key easily in your DeepSeek account. Go to the API key settings. This is usually in your profile or account settings. There, you will find the option to create a new API key. Remember, when you reset your API key, all applications using the old key must be updated.
Can DeepSeek API be Integrated with Other AI Tools?
The compatibility of the DeepSeek API with other AI tools depends on the features and settings they support. DeepSeek wants to offer a flexible API. However, it is important to look at their documentation or reach out to their support team to confirm if it works with the specific AI tools you want to connect.
What are the Rate Limits for DeepSeek API?
The DeepSeek API has rate limits to keep usage fair and stop abuse. These limits depend on your subscription level and the model you choose. You can find full details about the rate limits and usage rules in their documentation or by contacting their support team.