Master React JS Swag Shop Setup: Expert Guide
- Authors
- Name
- Geeks Kai
- @KaiGeeks
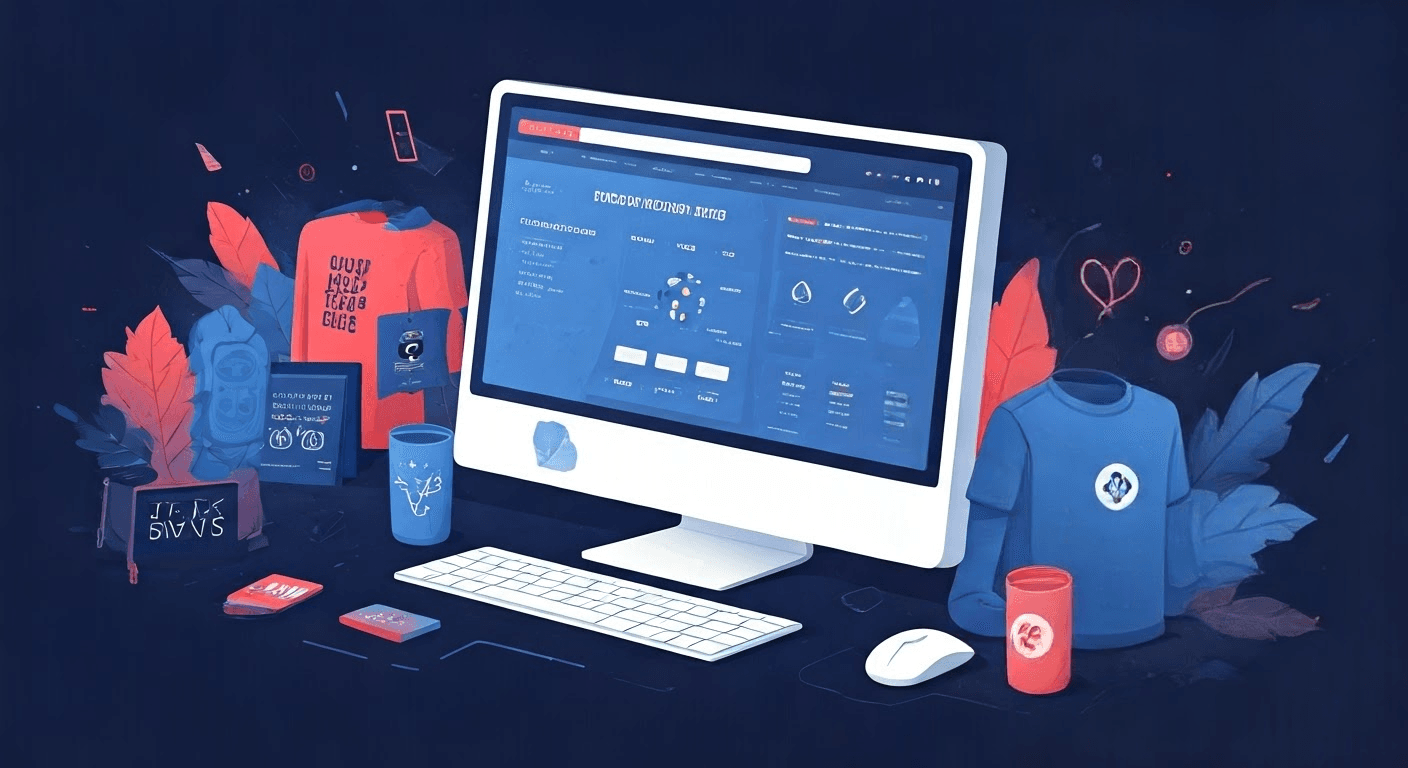
Key Highlights
- Quick and Easy Setup: Find out how to set up a swag shop using React JS without much trouble.
- Engaging Shopping Experience: Learn tips to build a friendly interface for your shop.
- Mobile-First Design: Make sure your swag shop looks good on all devices using responsive design methods.
- Streamlined Checkout Process: Look into ways to improve the checkout process for your customers.
- Secure and Reliable: Use the best practices to keep customer data safe and ensure your shop is secure.
Introduction
In today's digital world, a swag shop is important for any brand that wants to connect with its audience. This guide shows you how to create a working swag shop using React JS. React JS is a well-known library for making user-friendly and interactive websites. Whether you are just starting in web development or you have experience, this guide will help you learn what you need to know. You can use these tools to start your own React app swag shop.
Starting Your React JS Swag Shop
Before we go into the technical details, let's look at the essential steps to start your React JS swag shop. First, you need to choose the right tools and framework for your shop. React has a component-based structure. This makes it simple to create UI elements that you can use again, which helps in development.
Basic Project Setup
# Install Create React App globally
npm install -g create-react-app
# Create a new React project
npx create-react-app swag-shop
# Navigate to project directory
cd swag-shop
# Start the development server
npm start
Project Structure
swag-shop/
├── public/
│ ├── index.html
│ └── manifest.json
├── src/
│ ├── components/
│ │ ├── ProductList.js
│ │ ├── ProductCard.js
│ │ ├── Cart.js
│ │ └── Checkout.js
│ ├── pages/
│ │ ├── Home.js
│ │ ├── Product.js
│ │ └── Cart.js
│ ├── App.js
│ └── index.js
└── package.json
Sample Product Component
import React from 'react';
const ProductCard = ({ product }) => {
return (
<div className="product-card">
<img src={product.image} alt={product.name} />
<h3>{product.name}</h3>
<p className="price">${product.price}</p>
<button onClick={() => addToCart(product)}>
Add to Cart
</button>
</div>
);
};
export default ProductCard;
Designing Your Swag Shop's User Interface
A good-looking and easy-to-use interface is very important for any online shop. When you are building your React JS swag shop, focus on making it simple, clear, and fun for your users. Start by dividing your shop into logical parts like a product listing page, individual product detail pages, and a shopping cart page.
Responsive Grid Layout Example
.product-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 2rem;
padding: 2rem;
}
@media (max-width: 768px) {
.product-grid {
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
gap: 1rem;
padding: 1rem;
}
}
Shopping Cart Implementation
import React, { useState } from 'react';
const Cart = () => {
const [items, setItems] = useState([]);
const removeItem = (id) => {
setItems(items.filter(item => item.id !== id));
};
const updateQuantity = (id, quantity) => {
setItems(items.map(item =>
item.id === id ? { ...item, quantity } : item
));
};
return (
<div className="cart">
{items.map(item => (
<div key={item.id} className="cart-item">
<img src={item.image} alt={item.name} />
<div className="item-details">
<h4>{item.name}</h4>
<p>${item.price}</p>
<input
type="number"
value={item.quantity}
onChange={(e) => updateQuantity(item.id, e.target.value)}
min="1"
/>
</div>
<button onClick={() => removeItem(item.id)}>Remove</button>
</div>
))}
</div>
);
};
export default Cart;
Tips for Crafting an Engaging Shopping Experience
- Intuitive Navigation: Make sure users can easily move between different parts of your shop. This way, they can navigate without getting confused. Use a clear menu, show where they are in the shop with breadcrumbs, and add a search bar for finding products quickly.
- Compelling Visuals: Use high-quality images of your swag taken from different angles. You might also add product videos or 360-degree views for a better experience. Use white space wisely to keep the layout neat and visually pleasing.
- Personalized Recommendations: Create a system that suggests products based on what users have browsed or bought before. This personal touch can help connect with users and encourage them to buy more.
Implementing Responsive Design for All Devices
With more people using mobile devices to shop online, making sure your swag shop works well on all screens is very important. Responsive web design helps your website fit easily to different screen sizes. This way, it offers a great experience for viewing and interacting on any device.
Conclusion
In conclusion, starting a React JS Swag Shop needs careful planning and execution. You should pick the right tools and make your user interface easy to use. This helps create a fun shopping experience for your customers. It is also important to manage your products well, use secure payment options, and improve the checkout process. These steps are vital for building a strong online store.
Frequently Asked Questions
How Do I Manage State in a React JS Swag Shop?
Managing state in a React JS swag shop means dealing with changing data easily. This includes product info, shopping cart items, and how users interact with the app. You can use JavaScript libraries like Redux or Zustand. You can also use React's built-in Context API to handle your app's state well.
Can I Integrate Payment Gateways in React JS?
Yes, you can add payment gateways like Stripe to your React JS swag shop. These gateways offer APIs. They help you safely process transactions. You can also manage checkout processes and pricing details in your application.
What Are the Best Practices for Product Management?
Efficient product management is all about arranging and showing your swag in a smart way. You can use React components to build product cards that you can use over and over. Bring in an API to get inventory data from a database. Make an easy interface for handling product details.
How to Ensure Security for Customer Data?
- Protecting customer data is very important.
- Make sure your website uses HTTPS for safe communication.
- Use strong API authentication to keep sensitive areas safe.
- Encrypt important data stored in your database.
- Follow rules for data privacy.
Tips for Enhancing the Checkout Process in React JS
To make the checkout experience better, you should:
- Simplify your forms.
- Use React components to design a clear checkout process.
- Offer real-time order updates.
- Include a guest checkout option.
- Use notifications for a smooth shopping cart and checkout experience.