- Published on
AI Image Reader: Extracting Financial Data Made Easy
- Authors
- Name
- Geeks Kai
- @KaiGeeks
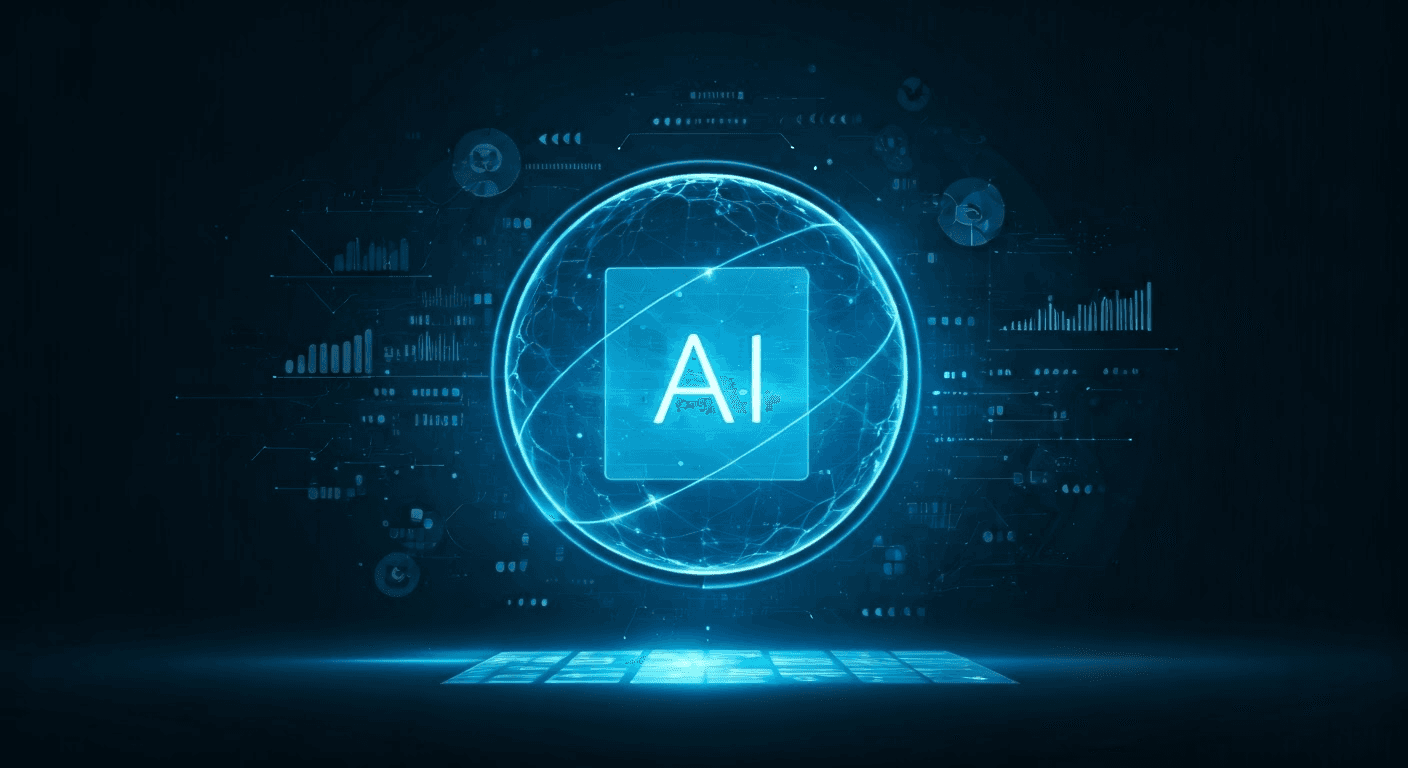
Key Highlights
- AI is revolutionizing financial data extraction, offering a faster and more accurate alternative to manual methods
- AI-powered image readers use OCR and deep learning to process financial documents like invoices, receipts, and bank statements
- This technology streamlines financial operations, reduces errors, and frees up human resources for more strategic tasks
- Real-world applications include financial statement analysis, fraud detection, and risk management
- Businesses can choose from various AI-powered image reader solutions, selecting the one that best suits their needs and budget
Introduction
The financial sector relies heavily on data. Getting useful insights from this data is very important for success. In the past, this process took many hours of manual work, which often led to mistakes and waste. Now, with the rise of artificial intelligence (AI) and advanced optical character recognition (OCR) technology, we are changing how we extract data from financial documents. This blog looks at how AI-driven image readers are changing the game for financial data extraction.
The Evolution of Financial Data Extraction
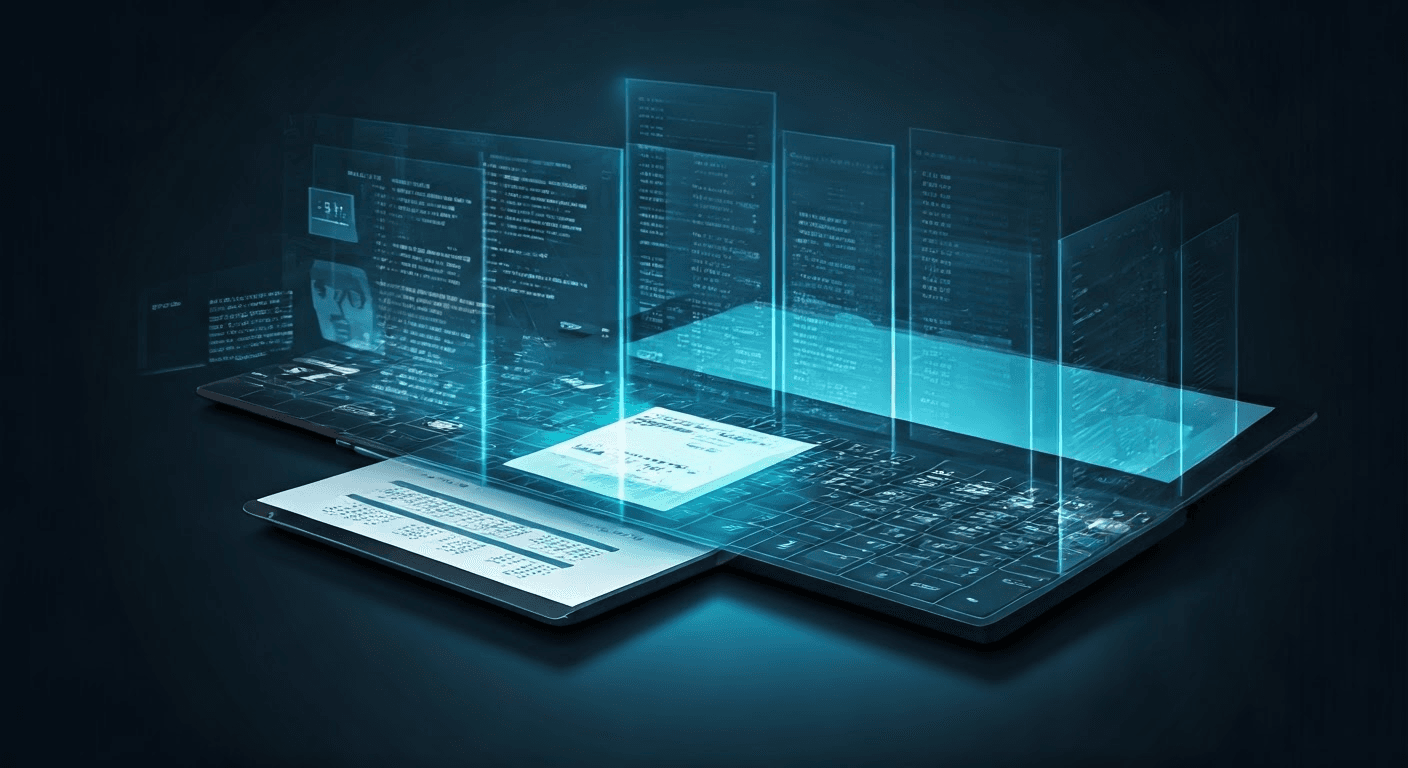
In the past, getting financial data was a slow and careful process. People had to spend a lot of time looking at bank statements, financial reports, and invoices. They would manually enter information into spreadsheets. This job was dull and often didn't go well. Mistakes could happen, which might lead to wrong financial reports and analyses.
Now, with the integration of AI into financial operations, things have changed. We see much better efficiency and accuracy in data extraction.
From Manual to Automated: The Shift in Data Processing
Traditionally, manual data entry was very important for processing financial data. However, this method had many problems. The repetitive work of data entry often made people tired, which led to mistakes. These mistakes could cause issues, resulting in incorrect financial records and reports.
Here's a simple example of how we can use Python with Tesseract OCR to extract text from financial documents:
import pytesseract
from PIL import Image
import cv2
import numpy as np
def preprocess_image(image_path):
# Read the image
img = cv2.imread(image_path)
# Convert to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Apply thresholding to preprocess the image
gray = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
# Apply dilation to connect text components
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3,3))
gray = cv2.dilate(gray, kernel, iterations=1)
return gray
def extract_text_from_image(image_path):
# Preprocess the image
processed_image = preprocess_image(image_path)
# Extract text using Tesseract
text = pytesseract.image_to_string(processed_image)
return text
def parse_financial_data(text):
# Add custom parsing logic for financial data
# This is a simple example - you'd want to add more sophisticated parsing
import re
# Find amounts
amounts = re.findall(r'\$\d+(?:\.\d{2})?', text)
# Find dates
dates = re.findall(r'\d{2}/\d{2}/\d{4}', text)
return {
'amounts': amounts,
'dates': dates
}
# Example usage
if __name__ == "__main__":
image_path = "financial_document.jpg"
extracted_text = extract_text_from_image(image_path)
financial_data = parse_financial_data(extracted_text)
print("Extracted Amounts:", financial_data['amounts'])
print("Extracted Dates:", financial_data['dates'])
Understanding AI's Role in Transforming Data Extraction
At the center of this change are smart AI algorithms. These are important in machine learning and deep learning. These algorithms help computers learn from large sets of financial documents. Here's an example of how to use deep learning for document classification:
import tensorflow as tf
from tensorflow.keras import layers, models
def create_document_classifier():
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(300, 300, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(4, activation='softmax') # For 4 document types
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Example document types: invoice, receipt, bank statement, financial report
document_types = ['invoice', 'receipt', 'bank_statement', 'financial_report']
Unveiling AI-Powered Image Readers for Finance
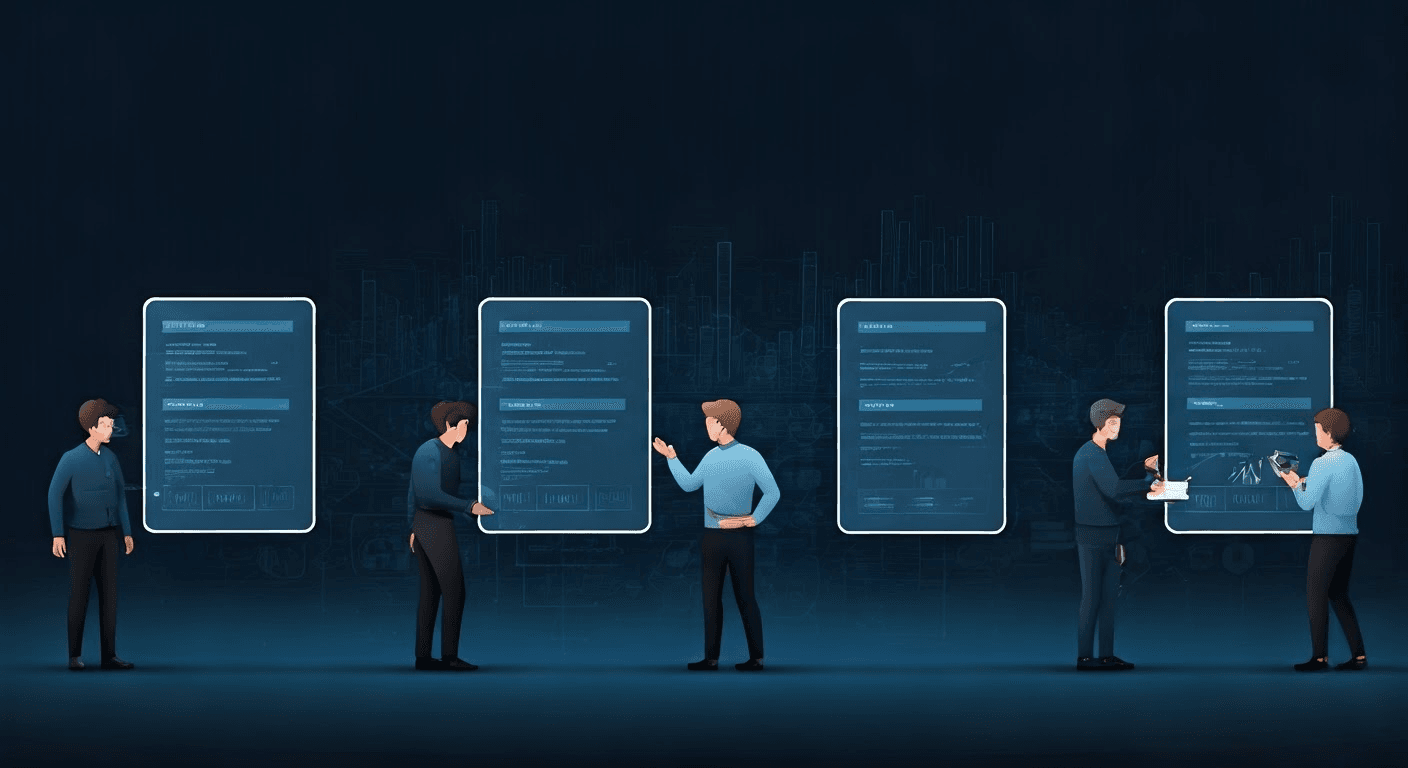
AI-powered image readers are a big step up in how we collect financial data. These systems do more than just basic OCR. They use smart algorithms to "understand" what is in financial documents and pull out data points with great accuracy.
Here's an example of how to integrate with a cloud-based OCR service (using Azure's Form Recognizer):
from azure.ai.formrecognizer import DocumentAnalysisClient
from azure.core.credentials import AzureKeyCredential
def analyze_financial_document(endpoint, key, document_path):
document_analysis_client = DocumentAnalysisClient(
endpoint=endpoint,
credential=AzureKeyCredential(key)
)
with open(document_path, "rb") as f:
poller = document_analysis_client.begin_analyze_document(
"prebuilt-document", document=f
)
result = poller.result()
# Extract key-value pairs
for kv_pair in result.key_value_pairs:
key = kv_pair.key.content if kv_pair.key else ""
value = kv_pair.value.content if kv_pair.value else ""
print(f"Key: {key}, Value: {value}")
# Extract tables
for table in result.tables:
print("Table found:")
for cell in table.cells:
print(f"Cell text: {cell.content}")
Key Benefits of Using AI for Financial Document Analysis
The use of AI in looking at financial documents offers many good benefits:
- Better Accuracy and Speed: AI image readers lower the chance of mistakes that happen with manual data entry
- Quicker Processing Times: By using AI for data extraction tasks, we can reduce processing time significantly
- Higher Quality Data: AI image readers turn unstructured data into a structured format
- Savings on Costs: When we automate data extraction tasks, we free up our people to work on important projects
Implementing AI Image Readers in Financial Operations
Here's a practical example of implementing an invoice processing system:
from transformers import LayoutLMv2Processor, LayoutLMv2ForTokenClassification
import torch
class InvoiceProcessor:
def __init__(self):
self.processor = LayoutLMv2Processor.from_pretrained("microsoft/layoutlmv2-base-uncased")
self.model = LayoutLMv2ForTokenClassification.from_pretrained("microsoft/layoutlmv2-base-uncased")
def process_invoice(self, image_path):
# Load and preprocess image
image = Image.open(image_path).convert("RGB")
encoded_inputs = self.processor(
image,
return_tensors="pt",
padding="max_length",
truncation=True
)
# Make prediction
outputs = self.model(**encoded_inputs)
predictions = outputs.logits.argmax(-1).squeeze().tolist()
# Process predictions
tokens = self.processor.tokenizer.convert_ids_to_tokens(encoded_inputs["input_ids"].squeeze().tolist())
return self._extract_invoice_data(tokens, predictions)
def _extract_invoice_data(self, tokens, predictions):
# Add custom logic to extract specific fields like:
# - Invoice number
# - Date
# - Amount
# - Vendor details
pass
# Example usage
invoice_processor = InvoiceProcessor()
result = invoice_processor.process_invoice("invoice.jpg")
Real-World Applications: AI in Financial Statement Analysis
AI image readers have proven incredibly valuable in various financial processes. Here's a table of key financial metrics that can be automatically extracted:
Information | Description |
---|---|
Revenue | Total income generated |
Gross Profit | Revenue minus the cost of goods sold |
Operating Expenses | Costs incurred through normal business operations |
Net Income | Profit after all expenses are deducted from revenue |
Conclusion
AI-powered image readers are changing how we extract financial data. They make the process faster and more accurate. Moving from manual work to automated data processing has made operations easier and better for decision-making in finance.
Frequently Asked Questions
Can AI image readers extract data from handwritten financial documents?
Yes, many advanced AI image readers use OCR to pull text from handwritten documents. However, accuracy can vary depending on the handwriting clarity and AI sophistication.
How do AI-powered image readers ensure data privacy and security?
AI-powered image readers prioritize data privacy and security through:
- Encryption
- Access controls
- Secure data storage
- Regular security audits
What are the limitations of AI in financial data extraction?
While powerful, AI has some limitations:
- Complex layouts can be challenging
- Multiple document formats may cause issues
- Multi-language documents require specialized models
- Handwriting recognition isn't perfect