Utilizing NavLink for State Passing in React Router
- Authors
- Name
- Geeks Kai
- @KaiGeeks
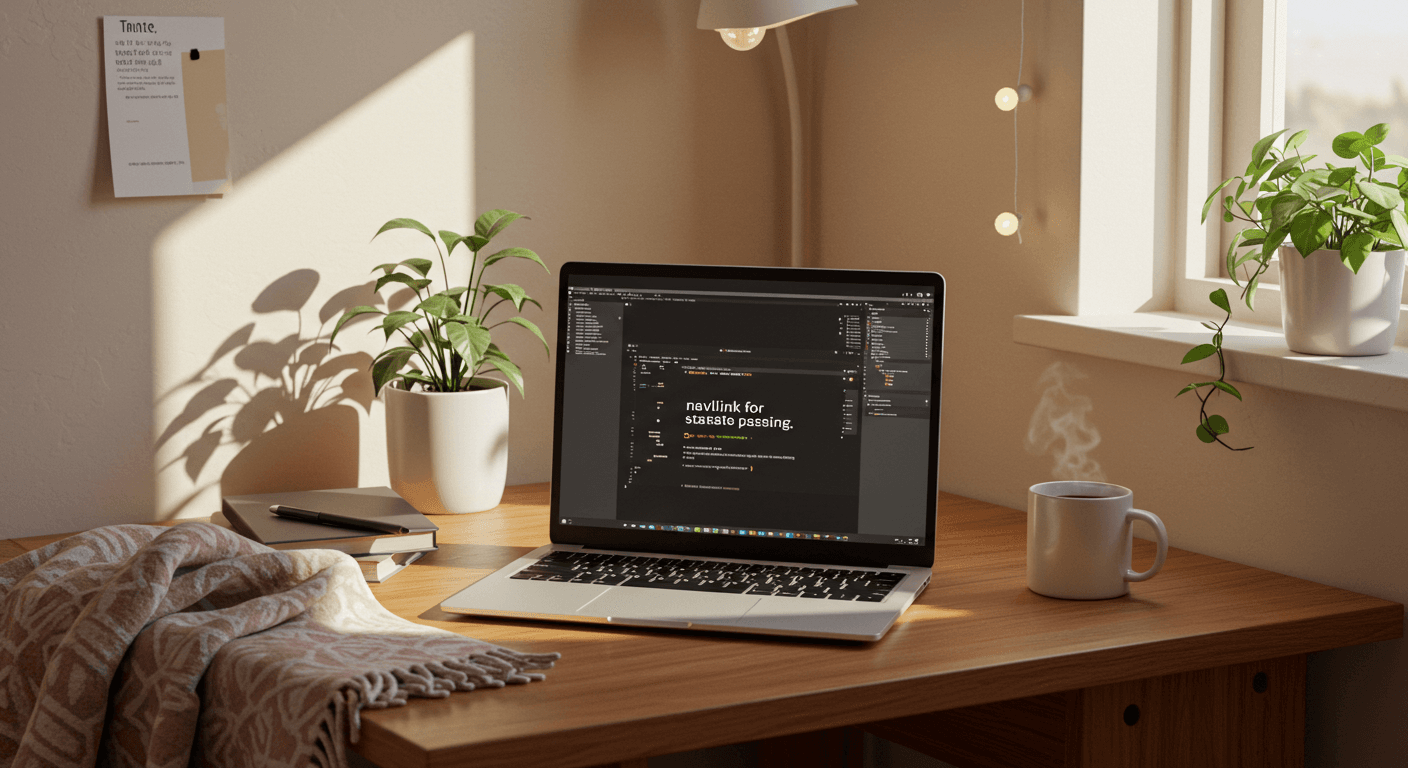
Key Highlights
- The NavLink component from React Router allows easy navigation in single-page apps
- You can easily send data using state props with NavLink
- To access the sent data in the target component, use the
useLocation
hook - Style active NavLinks to give clear feedback to users
- When you master NavLink, you can make navigation in your React projects simple and user-friendly
Introduction
In the world of React, it is very important to manage navigation in single-page applications well. This is where React Router helps you out. This blog post will look closely at a part of React Router called NavLink
. We will see how to use NavLink to move between different components. We will also learn how to pass data during these transitions. This makes your React applications more dynamic and easier for users.
Understanding React Router and NavLink
Before we get into the details of how to implement things, let's first understand the roles that React Router and NavLink have in a React application.
The Role of React Router in Single Page Applications
React Router gives navigation power to your single-page applications (SPAs). Think of it like making a multi-page website but inside one HTML document -- that's how SPAs work! React Router helps you set up different routes for your React app, with each route linked to a specific component. When the URL changes, like when a user clicks on a link, React Router shows the right component. This happens without leaving the same page, which makes the user experience smooth and fast.
Introduction to NavLink and Its Features
In React Router, NavLink is a special type of <a>
tag. It helps you navigate between different parts of your app. It also offers extra styling options. When you use NavLink instead of a regular <a>
tag, you get more features that are great for single-page applications. You can think of it as a clever link component that knows about your React Router routes. This makes it useful for navigation menus where you want to show which link is active.
Setting Up Your Environment
Now, let's dive into hands-on work! But before we write any code, let's make sure your React development environment is ready with bootstrap. We need to have all the right tools and libraries in place.
Required Tools and Libraries for React Development
To use React Router DOM well, make sure you have these things:
- Node.js and npm: These are key for your JavaScript development. They help you manage packages and run scripts.
- React Project: If you're starting from scratch, build a new React project with
create-react-app
. This will give you a basic React application set up with all the needed settings.
Installing React Router DOM
React Router DOM is the main library we will use to apply React Router in our web app. It's easy to install it using npm or yarn. Just go to your project's main folder and run this command:
npm install react-router-dom
Beginner's Guide to Using NavLink in React Router
Step 1: Creating Your React Application
Assuming you already have a React app running, let's go over the main structure of your App
component. If you haven't made a React app yet, you can start one quickly with Create React App by using these commands:
npx create-react-app my-react-app
cd my-react-app
Step 2: Implementing NavLink to Navigate Between Components
First, you need to import some important parts from react-router-dom. Here's a complete example of implementing NavLink:
import React from "react"
import { BrowserRouter, Routes, Route, NavLink } from "react-router-dom"
import Home from "./components/Home"
import About from "./components/About"
function App() {
return (
<BrowserRouter>
<div>
<nav>
<ul>
<li>
<NavLink to="/">Home</NavLink>
</li>
<li>
<NavLink to="/about">About</NavLink>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
</div>
</BrowserRouter>
)
}
export default App
Conclusion
In conclusion, learning how to use NavLink in React Router can greatly improve navigation in your apps. By getting to know React Router and NavLink, you can make the user experience better and keep information flowing smoothly. Using NavLink well allows you to pass state easily, making interactions more dynamic in your app. Keep in mind, with NavLink, you can increase the features of your React projects and give users a smooth and engaging browsing experience. So, dive in, try things out, and discover how NavLink can enhance your React Router applications.
Frequently Asked Questions
How do I pass state using NavLink in React Router?
To pass state, you need to use the state
prop in your NavLink
component. Here's an example:
<NavLink to="/profile" state={{ from: "homepage" }}>
Profile
</NavLink>
You can access this state data in the Profile
component.
Can NavLink be used to navigate to external links?
No, NavLink
is meant for moving around inside your React app. If you want to link to an external URL, it's better to use a simple <a>
tag with the href
attribute.
What is the difference between NavLink and Link in React Router?
Both NavLink
and Link
help with navigation in a React Router app. The main difference is that NavLink
provides extra styling options for when a link is active. This makes NavLink
perfect for navigation menus.
How can I set an active class using NavLink?
You can style the active NavLink
easily with CSS. Just focus on the active
class. This class is added automatically to the active NavLink
. For example, you can use: .active { font-weight: bold; }
.
Is it possible to pass functions as state in NavLink?
It's usually not a good idea to send functions directly as state. If you want to share a function, think about sharing a reference to it instead. This way, the component that gets it can call the function when it needs to.